In software development, it is common practice to sign software packages to prove their authenticity. In the same way, it is possible to sign Git commits and tags, usually with GPG, to prove that the code came from you and that it wasn’t maliciously made or altered by an attacker using your identity.
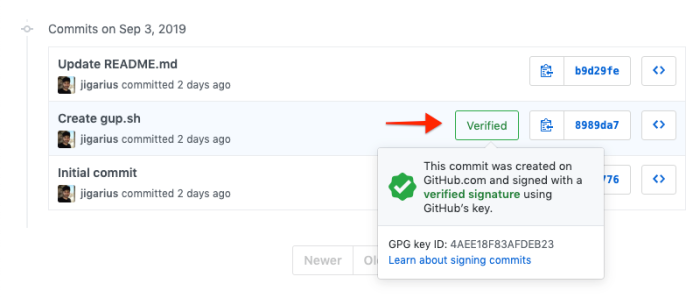
The above screenshot shows how a signed commit appears on GitHub to let your team members know that it came from the right person.
Two Minute Version
- You must have
gpg
installed. If not, you install it first. - Generate a GPG key with
gpg --gen-key
orgpg --full-gen-key
. - List your GPG keys with
gpg --list-secret-keys --keyid-format long
and get the part after the/
in the line that starts withsec
. For example:0E6198DFB2D67A26
. - Run
gpg --armor --export 0E6198DFB2D67A26
, copy the output, and configure it on the remote repository, e.g. GitHub, Gitlab, etc. - Configure Git to use the selected key for signing commits:
git config user.signingkey 0E6198DFB2D67A26
.- Use the
--global
flag to configure git globally.
- Use the
- Create signed commits by adding the
-S
parameter to your commits. For example,git commit -S -m "Hello world."
Step 1: Installing GPG
GPG is a free encryption software which can be used to encrypt and decrypt files. We will use it to sign our Git commits and tags. On a UNIX-like operating systems like Ubuntu and MacOS, gpg
usually comes pre-installed. For windows, you have to download and install GPG yourself. You can test your GPG installation and version with the following command:
$ gpg --version
gpg (GnuPG) 2.2.16
If gpg
doesn’t work for you, then try writing gpg2
instead and see if that works. If the gpg2 command works for you, you can tell Git to use it for signing commits with the following command:
git config --global gpg.program gpg2
As a matter of fact, Git doesn’t care what program you use for signing your commits as long as it works like GPG.
Step 2: Configuring GPG keys
Generating GPG keys
With GPG installed, we now need to generate a pair of keys – similar to what we do with SSH keys. The following command will guide you through the GPG key creation process.
# For lesser options, use: gpg --gen-key.
#
# You can safely use the following settings:
# Key type: RSA and RSA.
# Size of key: 4096 bits.
# Validity of key: zero (unless you want your key to expire).
gpg --full-gen-key
Next, GPG will ask for the following details to establish your identity. Here’s an example of the information GPG will ask for:
GnuPG needs to construct a user ID to identify your key.
Real name: Johnny Bravo
Email address: johnny.bravo@example.com
Comment: # Optional.
If you are planning to use different email addresses on different projects, you’ll have to generate one GPG key for each email address.
GPG will also ask you to create a password for the key – You’ll be prompted for this password whenever you try to use your key. I’d recommend choosing a strong and memorable password.
In order to put your GPG keys to use, you will need access to your key ID and the public key. We’ll see how to get those in the sections below.
Listing GPG keys
Once you have setup a number of GPG keys, you might want to see a list of all your keys. You can do that with the following command:
# List all GPG public keys.
$ gpg --list-keys --keyid-format long
# List all GPG secret keys.
$ gpg --list-secret-keys --keyid-format long
For our example in this tutorial, we’ll only see the keys for a particular identity. We do this by appending an email address to the end of one of the above commands:
# Append an email address to filter keys by email.
$ gpg --list-secret-keys johnny.bravo@example.com
sec rsa4096/0E6198DFB2D67A26 2019-09-05 [SC]
CD1EA7BE24508E01E47010DB0E6198DFB2D67A26
uid [ultimate] Johnny Bravo <johnny.bravo@example.com>
ssb rsa4096/0AA338E3ABA6930F 2019-09-05 [E]
If you run the command without the email parameter, you will see all your GPG keys.
Getting GPG key ID
To get the ID of your GPG key, use the command above to see a list of keys first. Now, focus on the line that says sec
, i.e. rsa4096/0E6198DFB2D67A26
. The part after the slash (/
) is the GPG key ID. For example: 0E6198DFB2D67A26
.
Getting GPG public key
To see the public key, you need to have a key ID as mentioned above. Use the following command to see your full public key:
# The syntax is: gpg --armor --export KEY-ID
$ gpg --armor --export 0E6198DFB2D67A26
-----BEGIN PGP PUBLIC KEY BLOCK-----
mQINBF1xnckBEADIeAmeXUAtUJ5EHr/xwpzNU1C/NixbaHnmFhgnMa076OpbJxvP
kpOGciSN9a4xn39soxFY56G3rO3R7ecANBXjsTi+sz4CzKxU6OH2Eu1tJnidLVg2
# ...
aPA1Ij+YjJ+2QOcFDU0+fSTYv+SYAmLsmDK9Fqib9yUjTQgTau8hslBS3YhzlAxK
szXI7gyqWSwNWbvkpJtnR/1eLh/CRC5pFX62AvpnJbqnistNY8OpYCV+kzvwjEiL
=gAQS
-----END PGP PUBLIC KEY BLOCK-----
Deleting GPG Keys
If you ever want to remove a public or private key, you need to run one of the following commands depending on your needs:
# Deletes GPG public key.
$ gpg --delete-key johnny.bravo@example.com
# Deletes GPG secret key.
$ gpg --delete-secret-key johnny.bravo@example.com
Step 3: Configuring Git
Now, we need to tell Git about our GPG keys to be able to sign and verify things.
Add GPG keys to Git repository manager
Most Git repository managers like GitHub, GitLab and BitBucket provide an option to add GPG public keys to your account. The option to add your GPG public key to your Git repo manager is usually under profile settings.
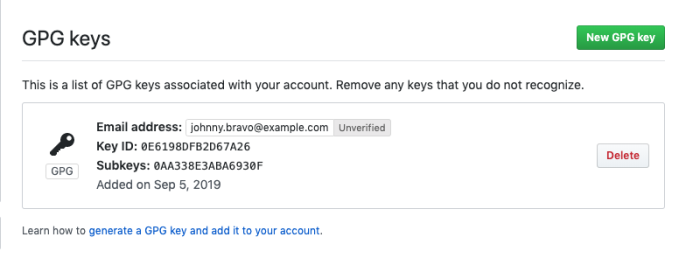
Run the command gpg --armor --export KEY-ID
to get your GPG public key and add it to your repository manager. These keys are then used to generate badges to indicate if your commits are verified. This lets your team members easily check if your commits are signed and hence, authentic.
Add GPG keys to Git command-line tool
Use the following command to tell your command-line tool to use a specific GPG key for signing your commits:
# The syntax is git config user.signingkey KEY-ID
git config user.signingkey 0E6198DFB2D67A26
You can use git config --global user.signingkey KEY-ID
to save this in your global Git settings which will then be used for all projects.
Step 4: Signing
Now that the GPG keys are in place, it’s actually time to sign commits and tags!
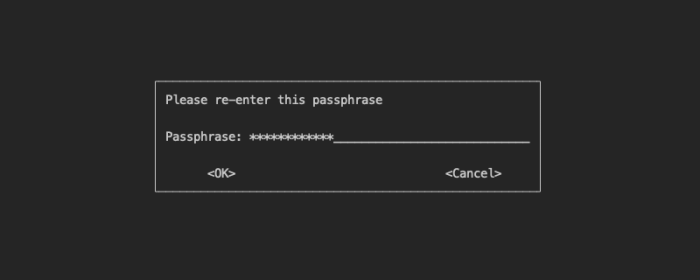
Signing Git commits
To create a signed commit, add the additional parameter -S
to your git commit
command like this:
git commit -S -m "Enough about you, let's talk about me, Johnny Bravo."
Doing this will show you a dialog where you will have to enter your GPG password to sign and make the commit.
Signing Git tags
To create a signed tag, add the additional parameter -s
to your git tag
command like this:
git tag -s v1.19
Doing this will show you a dialog where you will have to enter your GPG password to sign and make the commit.
Always sign Git commits
If you’ve decided that you always want to sign your commits and tags, then you can update your git configuration accordingly with the following command:
# Enable signing for the project.
$ git config commit.gpgsign true
# Enable signing globally.
$ git config --global commit.gpgsign true
Conclusion
- Signing GPG commits is an extra layer of security that help verify if a commit or a tag was actually made by you.
- It is fairly easy to sign Git commits with GPG – all you need to do is generate a key and configure it with Git.
- Signed git commits usually have a “verified” badge on Git repository managers like GitHub, GitLab, BitBucket, etc.
Next steps
- Try making signed commits to get the cool verified badge.
- Read more about GNU Privacy Guard (GPG).
- Leave comments to tell us about your experience.